Examples - Sensorboard - Compass
Compass
This Example shows the use of a digital Compass.
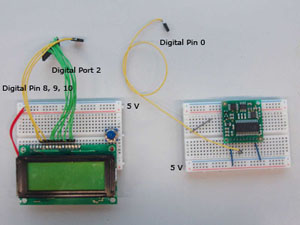
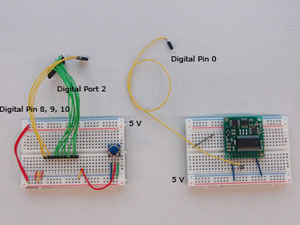
Source - Main
/************************************************************************************************** * * Compass CMPS03 * * Version: 1.0.0 - September 2008 * Author: Etienne Ribeiro / tutorial assistant caad / eribeiro[at]ethz.ch * Supervisor: Christoph Wartmann / chair for caad - ETH Zürich / wartmann[at].arch.ethz.ch * * Desc: Shows the use of the CMPS03 Compass Module. * ***************************************************************************************************/ // Const static int digitalpin = 0; static long maxImpules = 40000; static boolean enableSerial = false; // Setup void setup() { // LED (2 * blink) pinMode(48, OUTPUT); digitalWrite(48, HIGH); delay(200); digitalWrite(48, LOW); delay(200); digitalWrite(48, HIGH); // Serial (Print to serial if enableSerial = true) if (enableSerial == true) Serial.begin(9600); // Pin Mode pinMode(digitalpin, INPUT); } void loop() { // Impuls (mesure 2 times) long ImpulesLength = pulseIn(digitalpin, LOW); ImpulesLength = pulseIn(digitalpin, HIGH); long tmpImpulesLength = pulseIn(digitalpin, LOW); tmpImpulesLength = pulseIn(digitalpin, HIGH); if (tmpImpulesLength > ImpulesLength) ImpulesLength = tmpImpulesLength; // LCD Display_Clear(); int num = (double)ImpulesLength / (double)maxImpules * (double)8; switch(num) { case 0: case 4: Display_Println(" ||"); Display_Println(" ||"); Display_Println(" ||"); break; case 1: case 5: Display_Println(" //"); Display_Println(" //"); Display_Println(" //"); break; case 2: case 6: Display_Println(""); Display_Println(" ==="); Display_Println(""); break; case 3: case 7: Display_Println(" \\"); Display_Println(" \\"); Display_Println(" \\"); break; } Display_Println(" ", ImpulesLength / 1000); // Debug if (enableSerial == true) { Serial.print(ImpulesLength, DEC); Serial.println(""); } // Wait delay(100); }
Source - LCD-Display
/************************************************************************************************** * * LCD Class * * Version: 1.0.0 - September 2008 * Author: Etienne Ribeiro / tutorial assistant caad / eribeiro[at]ethz.ch * Supervisor: Christoph Wartmann / chair for caad - ETH Zürich / wartmann[at].arch.ethz.ch * * Desc: Library for an 14 Pin LCD Displays. * ***************************************************************************************************/ #include <LiquidCrystal.h> // CONST: const int port = 2; // Port on Wiring Board const int pins[] = {8, 9, 10}; // Pins on Wiring Board // VAR LiquidCrystal myDisplay = LiquidCrystal(pins[0], pins[1], pins[2], port); int iLine = 0; // Methodes void Display_Clear(){ iLine = 0; myDisplay.clear(); myDisplay.home(); } void Display_Println(char str[]){ myDisplay.home(); myDisplay.setCursor(0, iLine); myDisplay.print(str); iLine += 1; } void Display_Println(char str[], char str2[]){ myDisplay.home(); myDisplay.setCursor(0, iLine); myDisplay.print(str); myDisplay.print(" "); myDisplay.print(str2); iLine += 1; } void Display_Println(char str[], int zahl){ myDisplay.home(); myDisplay.setCursor(0, iLine); myDisplay.print(str); myDisplay.print(" "); myDisplay.print(zahl); iLine += 1; } void Display_Print(char str[]){ myDisplay.print(str); }