Examples - Sensorboard - Supersonic
Supersonic
This Example shows the use of an ultrasonic sensor.
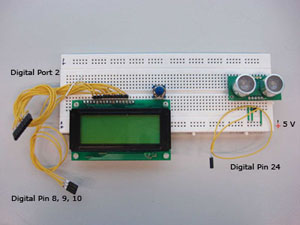
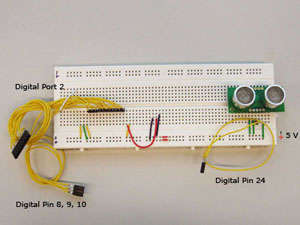
Source - Main
/************************************************************************************************** * * Supersonic SRF05 * * Version: 1.0.0 - September 2008 * Author: Etienne Ribeiro / tutorial assistant caad / eribeiro[at]ethz.ch * Supervisor: Christoph Wartmann / chair for caad - ETH Zürich / wartmann[at].arch.ethz.ch * * Desc: Shows the use of the SRF05 Supersonic Sensor on digital pin. * ***************************************************************************************************/ // Const static int digitalPin = 34; static boolean enableSerial = false; // (Var) int lastImp = 0; // Setup void setup() { //LED (2 * blink) pinMode(48, OUTPUT); digitalWrite(48, HIGH); delay(200); digitalWrite(48, LOW); delay(200); digitalWrite(48, HIGH); // LCD Display_Clear(); Display_Println("Supersonic LCD"); // Serial (Print to serial if enableSerial = true) if (enableSerial == true) Serial.begin(9600); pinMode(digitalPin, INPUT); } // Loop void loop() { // Trigger pulse digitalWrite(digitalPin, HIGH); delayMicroseconds(10); digitalWrite(digitalPin, LOW); // Mesure echo pulse int imp = pulseIn(digitalPin, HIGH); // LCD if (imp != lastImp) { lastImp = imp; Display_Clear(); Display_Println(" ", imp / 58, " cm"); } // Serial if (enableSerial == true) { Serial.print(imp); Serial.print(" -> "); Serial.print(imp / 58); Serial.println("cm"); } // Wait delay(50); }
Source - LCD Class
/************************************************************************************************** * * LCD Class * * Version: 1.0.0 - September 2008 * Author: Etienne Ribeiro / tutorial assistant caad / eribeiro[at]ethz.ch * Supervisor: Christoph Wartmann / chair for caad - ETH Zürich / wartmann[at].arch.ethz.ch * * Desc: Library for an 14 Pin LCD Displays. * ***************************************************************************************************/ #include <LiquidCrystal.h> // CONST: const int port = 3; // Port on Wiring Board const int pins[] = {37, 38, 39}; // Pins on Wiring Board // VAR LiquidCrystal myDisplay = LiquidCrystal(pins[0], pins[1], pins[2], port); int iLine = 0; // Methodes void Display_Clear(){ iLine = 0; myDisplay.clear(); myDisplay.home(); } void Display_Println(char str[]){ myDisplay.home(); myDisplay.setCursor(0, iLine); myDisplay.print(str); iLine += 1; } void Display_Println(char str[], char str2[]){ myDisplay.home(); myDisplay.setCursor(0, iLine); myDisplay.print(str); myDisplay.print(" "); myDisplay.print(str2); iLine += 1; } void Display_Println(char str[], int zahl){ myDisplay.home(); myDisplay.setCursor(0, iLine); myDisplay.print(str); myDisplay.print(" "); myDisplay.print(zahl); iLine += 1; } void Display_Println(char str1[], int zahl, char str2[]){ myDisplay.home(); myDisplay.setCursor(0, iLine); myDisplay.print(str1); myDisplay.print(" "); myDisplay.print(zahl); myDisplay.print(str2); iLine += 1; } void Display_Print(char str[]){ myDisplay.print(str); }