PIR Motion Sensor SE-90
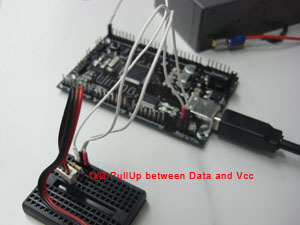
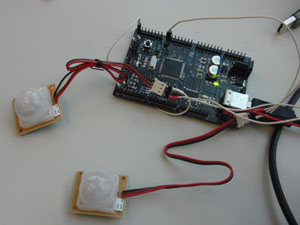
This Module can be used to plug several Motion-Sensors into your project. Connect your sensors to any digital pin on your board.
Download the source file
Table of contents
(top)
Source - modMotionPIRse10
/************************************************************************************************** * * PIR Motion Sensor SE-90 * * Version: 1.0.0 - January 2009 * Author: Etienne Ribeiro / tutorial assistant caad / eribeiro[at]ethz.ch * Supervisor: Christoph Wartmann / chair for caad - ETH Zürich / wartmann[at].arch.ethz.ch * * Desc: Shows the use of an analog Accelerator. This Module can be used to plug severel * Motion-Sensors to your project. Connect your sensors to any digital pin on your * board. * Note: Don't forget to connect a 10k Pull up resistor (connect vcc and outpin). * Note: The sensor needs up to 30 seconds after beeing powerd to return accurate * results. * ***************************************************************************************************/ // // Const int const MAX_SENSORS_TO_APPEND = 10; // // Var int mdt_digitalPin [MAX_SENSORS_TO_APPEND]; // Pin where PIR-Sensor's out pin is connected boolean mdt_isTransition [MAX_SENSORS_TO_APPEND]; int mdt_count = 0; long mdt_tmpMillis [MAX_SENSORS_TO_APPEND]; void MotionDetect_AppendSensor (int digitalPin) { mdt_isTransition[mdt_count] = true; mdt_tmpMillis [mdt_count] = 0; mdt_digitalPin[mdt_count] = digitalPin; pinMode(mdt_digitalPin[mdt_count], INPUT); mdt_count += 1; } int MotionDetect_isSensorFiring (int digitalPin, int waitMillisToEnd) { // get id (index in array) int id = mdt_getIdByPin (digitalPin); // return if pin has not been appended if (id == -1) return -1; // Something detected if (digitalRead(mdt_digitalPin[id]) == LOW) { mdt_tmpMillis[id] = millis(); if (mdt_isTransition[id] == true) { // go in only one time mdt_isTransition[id] = false; return 1; // "Something moved..." } } else { // Commit detection if nothing moved for TURN_OFF_AFTER-time if (mdt_isTransition[id] == false && mdt_tmpMillis[id] + waitMillisToEnd < millis()) { // go in only one time mdt_isTransition[id] = true; return 0; // "and stopped." } } // Return return -1; } // // Private Functions int mdt_getIdByPin (int digitalPin) { for (int id = 0;id<mdt_count;id++) { if (mdt_digitalPin[id] == digitalPin) return id; } return -1; }
(top)
Source - Main
/************************************************************************************************** * * PIR Motion Sensor SE-90 * * Version: 1.0.0 - January 2009 * Author: Etienne Ribeiro / tutorial assistant caad / eribeiro[at]ethz.ch * Supervisor: Christoph Wartmann / chair for caad - ETH Zürich / wartmann[at].arch.ethz.ch * * Desc: Shows the use of an analog Accelerator. This Module can be used to plug severel * Motion-Sensors to your project. Connect your sensors to any digital pin on your * board. * ***************************************************************************************************/ // // Const int digitalPin1 = 24; // Pin where PIR-Sensor's out pin is connected int digitalPin2 = 32; // // Var boolean initialized = false; // // Setup void setup() { // LED (2 * blink) pinMode(48, OUTPUT); digitalWrite(48, HIGH); delay(200); digitalWrite(48, LOW); delay(200); digitalWrite(48, HIGH); // Start Serial Serial.begin(9600); // Append Motion Sensor // You can append more Sensors if you want! MotionDetect_AppendSensor (digitalPin1); MotionDetect_AppendSensor (digitalPin2); } // // Loop void loop() { // if nothing moved during 3000 millis, sensor torns off and waits for next movement switch (MotionDetect_isSensorFiring (digitalPin1, 3000)) { case 1: Serial.println("Sensor1: Something moved..."); break; case 0: Serial.println("Sensor1: ...and stopped."); break; case -1: break; } switch (MotionDetect_isSensorFiring (digitalPin2, 3000)) { case 1: Serial.println("Sensor2: Something moved..."); break; case 0: Serial.println("Sensor2: ...and stopped."); break; case -1: break; } delay (200); }