Examples - Modules - Nokia LCD
Nokia LCD SGD-A
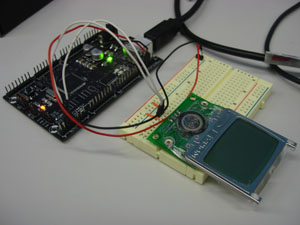
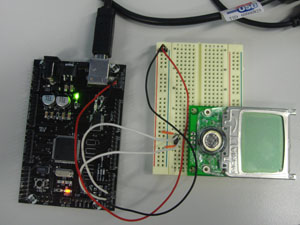
This Module shows the use of a Nokia LCD SGD-A. The Nokia LCD display has several additional futures, built in speaker, ram and eeprom. Connect your Display to SerialPort2 (Pin 2 and 3 on Wiring-Board). RxTx Pins are crossed so connect Rx to Tx and Tx to Rx.
Download the source file
Table of contents
(top)
Source - modNodiaLCD
/************************************************************************************************** * * Nokia LCD SGD-A * * Version: 1.0.0 - January 2009 * Author: Christoph Wartmann / chair for caad - ETH Zürich / wartmann[at].arch.ethz.ch * Etienne Ribeiro / tutorial assistant caad / eribeiro[at]ethz.ch * * Desc: This Module shows the use of a Nokia LCD SGD-A. The Nokia LCD display has several * additinal futures, build in speaker, ram and eeprom. Connect your Display to * SerialPort2 (Pin 2 and 3 on Wiring-Board). RxTx Pins are crossed so connect * Rx to Tx and Tx to Rx. * * Not implemented: - power management * - print Bitmaps * * Methodes: void startSerial () * Start communication. Must be called in setup() * void Wake() * If device is asleep, wake it up. (not tested) * void Sleep() * Tell device to go to sleep mode. (not tested) * void FirmwareRelease() * Prints firmware informations on screen. * void Ping() * Ping device. * void LCDContrast (int contrast) * Set contrast. (Usually not needed) * void LCDClear () * Clear Display. * void LCDLine (int x1, int y1, int x2, int y2) * Paint a line no display. * void LCDLineTo (int x, int y) * Line from display-pointer to next point. * void LCDPrint(char value[]) * Print a String. * void LCDPrint(int value) * Print a Number. * void LCDPrintln(char string[]) * Print a String and go to next line. * void LCDPrintln(int value) * Print a Number and go to next line. * // LCDBitmap * void LCDBitmap(byte buffer[]) * Print a Bitmap (not yet tested) * void SPKBeep () * Make Speaker beep. * void SPKBeep (int frequenceP, int frequenceE, int durantion_ms) * Make your own sound. * void RAM_Write (byte address, byte value) * Write to RAM. * int RAM_Read (byte address) * Read from RAM. * void EEPROM_Write (byte address, byte value) * Write to EEPROM. * int EEPROM_Read (byte address) * Read from EEPROM. * ***************************************************************************************************/ // // startSerial void startSerial () { Serial1.begin(9600); } // // Wake void Wake() { char result = 'Z'; do { Ping(); //delay(100); if(Serial1.available() > 0) result = Serial1.read(); } while(result != 'X'); } // // Sleep void Sleep() { Serial1.print('Z', BYTE); } // // FirmwareRelease void FirmwareRelease() { Serial1.print('F', BYTE); } // // Ping void Ping() { Serial1.print('X', BYTE); } // ************************************************************************** // // LCD // // // // LCDContrast // contrast: values from 0 to 127 // // Setting the contrast of LCD Display. void LCDContrast (int contrast) { if (contrast < 0) contrast = 0; if (contrast > 127) contrast = 127; Serial1.print('C', BYTE); Serial1.print(contrast, BYTE); } // // LCDClear // // Clear the display and set Pointer to 0,0 void LCDClear () { //Serial1.print('N'); Serial1.print('N', BYTE); //N } // // LCDLine // x1, y1, x2, y2 // // Print a line on display void LCDLine (int x1, int y1, int x2, int y2) { Serial1.print('P', BYTE); Serial1.print(x1, BYTE); Serial1.print(y1, BYTE); Serial1.print('L', BYTE); Serial1.print(x2, BYTE); Serial1.print(y2, BYTE); } // // LCDLineTo // x, y // // Print a line on display void LCDLineTo (int x, int y) { Serial1.print('L', BYTE); Serial1.print(x, BYTE); Serial1.print(y, BYTE); } // // LCDPrint // value: string / int / byte // // Print a line to display void LCDPrint(char value[]) { Serial1.print('S', BYTE); Serial1.print(strlen(value), BYTE); Serial1.print(value); } void LCDPrint(int value) { Serial1.print('S', BYTE); Serial1.print(intlen(value), BYTE); Serial1.print(value, DEC); } // // LCDPrintln // string // // Print a line to display void LCDPrintln(char string[]) { Serial1.print('S', BYTE); Serial1.print(strlen(string) + 1, BYTE); Serial1.print(string); Serial1.print("\n"); } void LCDPrintln(int value) { Serial1.print('S', BYTE); Serial1.print(intlen(value) + 1, BYTE); Serial1.print(value, DEC); Serial1.print("\n"); } // // LCDBitmap // string // // Print a line to display void LCDBitmap(byte buffer[]) { Serial1.print('S', BYTE); Serial1.print(strlen(buffer), BYTE); //Serial1.print(buffer); } // ************************************************************************** // // SPEAKER // // // // SPKBeep void SPKBeep () { SPKBeep (12, 249, 100); } // // SPKBeep // frequenceP: 1 - 16 // frequenceE: 0 - 255 // durantion_ms: 0 - 2065 void SPKBeep (int frequenceP, int frequenceE, int durantion_ms) { if (frequenceP < 1) frequenceP = 1; if (frequenceP > 16) frequenceP = 16; if (frequenceE < 0) frequenceE = 0; if (frequenceE > 255) frequenceE = 255; int t = durantion_ms / 8.1; if (t < 0) t = 0; if (t > 255) t = 255; Serial1.print('T', BYTE); Serial1.print(frequenceP, BYTE); Serial1.print(frequenceE, BYTE); Serial1.print(t, BYTE); delay (durantion_ms); } // ************************************************************************** // // SPEICHERN // // // // RAM_Write // address: 0 to 63 // value: 0 to 255 void RAM_Write (byte address, byte value) { if (address < 0) return; if (address > 63) return; if (value < 0) value = 0; if (value > 255) value = 255; Serial1.print('E', BYTE); Serial1.print(address, BYTE); Serial1.print(value, BYTE); } // // RAM_Read // address: 0 to 63 int RAM_Read (byte address) { // Send command if (address < 0) return -1; if (address > 63) return -1; Serial1.print('D', BYTE); Serial1.print(address, BYTE); // Read from Serial1 while(Serial1.available() == 0) { delay (10); } int val = Serial1.read(); return val; } // // EEPROM_Write // address: 0 to 127 // value: 0 to 255 void EEPROM_Write (byte address, byte value) { if (address < 0) return; if (address > 127) return; if (value < 0) value = 0; if (value > 255) value = 255; Serial1.print('W', BYTE); Serial1.print(address, BYTE); Serial1.print(value, BYTE); } // // EEPROM_Read // address: 0 to 127 int EEPROM_Read (byte address) { // Send command if (address < 0) return -1; if (address > 127) return -1; Serial1.print('R', BYTE); Serial1.print(address, BYTE); // Read from Serial1 while(Serial1.available() == 0) { delay (10); } int val = Serial1.read(); return val; } // // Private Functions int strlen(char value[]) { for (int i=0; i<=255; i++) { if (value[i]=='\0') return i; } } int strlen(byte value[]) { for (int i=0; i<=255; i++) { if (value[i]=='\0') return i; } } int intlen(int value) { for (int i=1; i<=5; i++) { if (floor(value / pow(10,i)) <= 0) return i; } }
(top)
Source - Main
/************************************************************************************************** * * Nokia LCD SGD-A * * Version: 1.0.0 - January 2009 * Author: Christoph Wartmann / chair for caad - ETH Zürich / wartmann[at].arch.ethz.ch * Etienne Ribeiro / tutorial assistant caad / eribeiro[at]ethz.ch * * Desc: This Module shows the use of a Nokia LCD SGD-A. The Nokia LCD display has several * additinal futures, build in speaker, ram and eeprom. Connect your Display to * SerialPort2 (Pin 2 and 3 on Wiring-Board). RxTx Pins are crossed so connect * Rx to Tx and Tx to Rx. * ***************************************************************************************************/ void setup() { // LED (2 * blink) pinMode(48, OUTPUT); digitalWrite(48, HIGH); delay(200); digitalWrite(48, LOW); delay(200); digitalWrite(48, HIGH); // Start Serial (Nokia LCD SGD-A is interfaced through Serial1 (or Serial)) startSerial(); // LCD /* // - Test contrast LCDContrast (70); */ // - Test Strings LCDClear (); // Clear LCD Display delay (60); LCDPrintln ("1 plus 1"); LCDPrint ("gibt "); LCDPrintln (1 + 1); LCDPrintln (""); LCDPrint ("1 + 2 gibt "); LCDPrint (1 + 2); LCDPrintln (""); /* // - Test Painting LCDClear (); // Clear LCD Display delay (60); LCDLine (30, 31, 42, 11); delay (60); LCDLine (43, 31, 52, 16); delay (60); LCDLineTo (20, 20); delay (60); LCDLineTo (34, 32); */ // SPEICHER /* // - Test Ram Serial.begin(9600); RAM_Write (10, 100); // address: 0 to 63, value: 0 to 255 int val = RAM_Read (10); // address: 0 to 63 Serial.println(val); // - Test EEPROM // (Don't use EEPROM if you don't need it! EEPROM can only be written limited times! //Serial.begin(9600); //EEPROM_Write (10, 100); // address: 0 to 63, value: 0 to 255 //int val = EEPROM_Read (10); // address: 0 to 63 //Serial.println(val); */ // SOUND /* // - Test Sound SPKBeep (); delay (300); SPKBeep (12, 249, 100);// frequenceP: 1 - 16, frequenceE: 0 - 255, durantion_ms: 0 - 2065 delay (300); SPKBeep (); */ /* // INFOS // - Print firmware release FirmwareRelease (); */ } void loop() { }